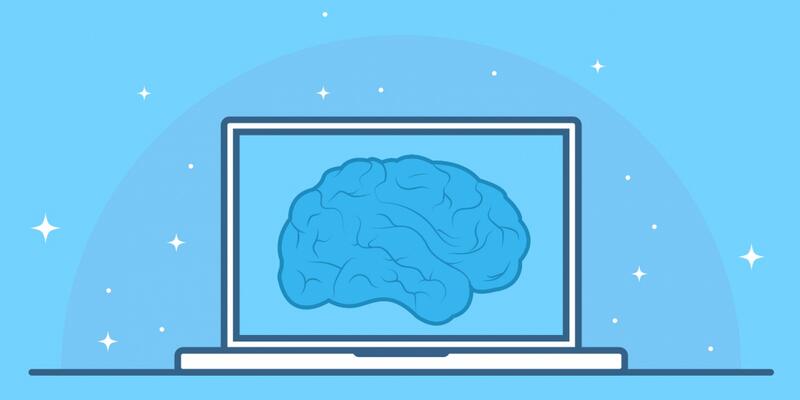
React's Fundamental Concepts
Ranking The Level of Concept Difficulty
React, the JavaScript library for creating user interfaces, has become the standard in professional front end.
This leads to a common problem: you want to learn React to help you as a JavaScript developer, but it's too much to learn. It helps to break the work down by difficulty level.
The topics to learn in React, and their difficulty, is roughly:
- General Structure: 5%
- Components: 5%
- Props: 10%
- State and Performance: 80%
You want to defer learning state until later, not as your first thing, because it's more difficult. If you can't, then avoid tutorials that messily mix components, props, and state.
Learn React Hooks
After React was debuted, there was a change in how React was written, involving state: an innovation called React hooks. You can use these to replace classes.
My advice would be to skip tutorials that have classes in them, and go straight for those structured more functionally, using hooks.
Target React tutorials that are built around hooks. Go back to classes after you've learned hooks.
For now this means skipping any beginner tutorial that has classes, or this in the code:
- class
- this
- bind
Or anything with code snippets like this:
class Example extends Component
Note that in any place where you can use classes, you can use hooks, and vice versa. This is really about ease of learning.
App Structure
Read up on how React apps are structured, and how their file structure tends to be set up.
But if you want a one-sentence description of a simple app: normally you have a /src folder with an App.js file that imports other files.
Components
Components are modular chunks of code, your basic React building block.
In your components you should be using JSX, which is basically 99% HTML and plain CSS, as far as it looks and is written.
To get started, try doing a basic React app w create-react-app and add components.
Props
Props are how you pass properties down to your components. Learn props.
Putting these together, with components and props, you now have enough to construct a very functional personal or portfolio site.
You could, and can, make dozens of sites with only this foundation.
Become Proficient with State
Concentrate on useState & how you use it in React, with hooks, or using functional components.
Without state, you can build a personal site, but you'll be very limited.
With a good working knowldge of state, not only can you include a submit form, but you'll have all the necessary functionality to build the interactive, responsive apps that define today's Web.
At this level you can also build an app that gets venture capital funding, attracts millions of users, and becomes a business.
Advanced Topics
After you've got a good grip on state, you can move on more advanced topics like useRef and memoization.
Beyond that, there’s also performance, how to bring down the load time by looking at things like bundle size.
Then there's further optimizations to improve performance and decrease cost, for which an expert consultant might be hired by a Facebook-grade company for maximum speed.
You can get very advanced with this, but you don’t need to know all that right at the beginning.
And that’s how you learn React - well enough to create apps and move on to more advanced topics.
Read the Official Docs
A good starting place is the official React docs, beginning with basic React concepts.
https://reactjs.org/docs/hello-world.html
You won't go wrong with these, starting from a strong foundation.
The React docs are reliable, accurate, and respected everywhere.
They also link to several courses, free and paid, which can easily fill your next month.
Start there, branch out, and in no time, you'll be up and running with React!