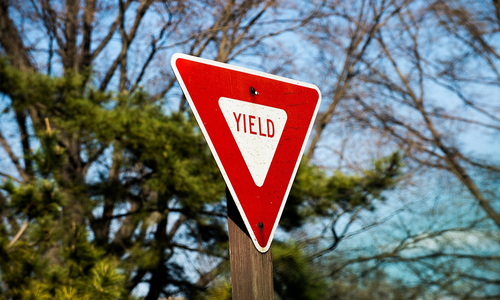
Using Yield in JavaScript
Explanation of Yield
Yield is a keyword that is used with, and only with, generator functions in JavaScript. You can think of it as a keyword to 'step' through functions.
A generator function is declared by appending your function with an asterisk, like so: function*.
function* number_maker
Inside of the generator function, you use yield.
How Generator Functions Work
Unlike 'normal' functions in JavaScript, a generator function returns a generator object.
Each time you call it, the generator function 'steps' through the function you defined. It does this again and again, each time you call it, until you run out of steps.
The yield keyword is used to define what each step of the generator function should return.
You can use the next() function with the iterator to get the next value object, and next().value to return the value itself.
Code Example
function* dungeon_room() {
yield "You are in a room."
yield "A monster attacks!"
yield "You win & live to fight another day."
}
const game=dungeon_room()
console.log(game.next().value)
console.log(game.next().value)
console.log(game.next().value)
console.log(game.next().value)
The generator function 'steps through' each line featuring the yield keyword and an expression.
This would print out each value, until there are no values left to print, at which point the value becomes undefined.
You are in a room.
A monster attacks!
You win & live to fight another day.
undefined