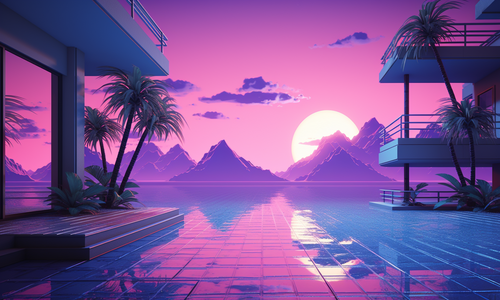
Using JavaScript with Rust Through Wasm
Why to Use JavaScript and WASM
JavaScript is everywhere on the web today. Rust is touted as a language of the future.
Through WASM, or WebAssembly, these two great languages can be used together.
Why? Because while JavaScript is reasonably fast, Rust, when compiled to WASM, is even faster, for many use cases.
For example, if you're writing a graphical application or a 3D game, JavaScript+Rust+WASM might be right for you.
What is WASM or WebAssembly
WASM is an assembly-like language which runs really fast in web browsers. The Rust code we'll be writing compiles down into it.
To quote a great blog post:
WebAssembly, commonly abbreviated as Wasm, is a portable binary intermediate language that can execute on different platforms in a virtual machine. The intermediate language is compiled... to the machine code appropriate for the architecture. Many programming languages compile to WebAssembly, including C, C++, C#, Rust, Go...
The Rust you write compiles into WASM, and that's what's run in your browser - and that code is often faster than anything you could've written in JavaScript directly. (Not always, but often.)
What is Rust
Rust a computer language created by Mozilla that describes itself as a general-purpose language which is "blazingly fast" and "memory efficient."
There is a hard emphasis on memory safety in the language. If your program is not memory safe, it won't compile (while similar code in other languages, like JavaScript, often would).
The plus side is that, once your code compiles and works, you can have greater confidence in it.
The Rust mascot is the crab.
How to Use JavaScript and WASM
The easiest way to get up and running with JavaScript, Rust and WASM is to clone an example project onto your computer.
We're going to use a simple example which adds two numbers together in Rust, and then compiles to WASM and runs in a JavaScript environment.
This example isn't really faster than the JavaScript version, but it shows you how to get WASM set up and what the process looks like.
You can start by using git clone to copy this wasm repository onto your computer.
git clone https://github.com/torch2424/wasm-by-example
Now navigate to the hello-world directory we'll be using for this example.
cd wasm-by-example/examples/hello-world/demo/rust
Look around this directory, to see how the index.html file, the index.js file, and the Rust code interrelate.
Run the command indicated in the README to prepare your files and compile the Rust down to WASM.
wasm-pack build --target web
The Rust files are compiled down to WASM, which is then accessed through your JavaScript file and rendered as usual on the HTML page.
To see this in action, you'll want to host the files locally. You can do this easily using Python's http server.
From within the hello-world/demo/rust folder, run this command:
python3 -m http.server
Normally, this will host your files on port 8000, and will serve up index.html by default.
So go to localhost:8000 in your browser.
You should see two numbers being added together, following the method described in the lib.rs file in the Rust directory.
#[wasm_bindgen]
pub fn add(a: i32, b: i32) -> i32 {
return a + b;
}
The matching JS code that makes it all work is shown below.
import wasmInit from "./pkg/hello_world.js";
const runWasm = async () => {
// Instantiate our wasm module
const helloWorld = await wasmInit("./pkg/hello_world_bg.wasm");
// Call the Add function export from wasm, save the result
const addResult = helloWorld.add(24, 24);
// Set the result onto the body
document.body.textContent = 'Hello World! addResult: ${addResult}';
};
runWasm();
Now you've run your first Rust WASM project.
To learn more, change the code and recompile it, then use Python's simple server to see your changes, live, in the browser.