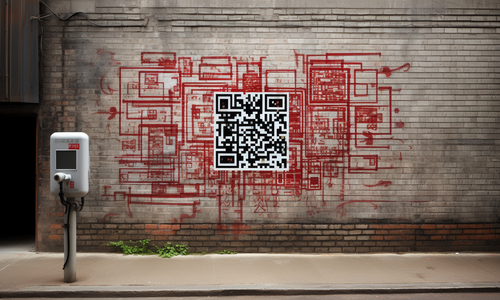
How to Create a QR Code Using JavaScript
You can create a QR code using JavaScript in just a few lines of code. From beginning to end, the process can be completed in about 5 minutes.
For this project, we'll be using the npm library QRious.
https://www.npmjs.com/package/qrious
QRious (that's how they spell it) is a JavaScript library for creating QR codes using HTML5 canvas.
Start by creating a new directory. I'll call mine qrsite.
mkdir qrsite
From within the directory, initialize a new npm project.
npm init -y
Install the QRious library with the npm install command.
npm install --save qrious
Now create the HTML and JS (JavaScript) to display your QR code.
If you want to do it all from within the HTML page, paste in the following code.
<!DOCTYPE html>
<html>
<body>
<canvas id="qr"></canvas>
<script src="node_modules/qrious/dist/qrious.js"></script>
<script>
(function() {
var qr = new QRious({
element: document.getElementById('qr'),
value: 'https://github.com/neocotic/qrious'
});
})();
</script>
</body>
</html>
In this example, the JavaScript code to generate the QR code is included inline, in the HTML page.
This QR code points to the GitHub website for the QRious library. If you take a picture of it with your phone, you'll see that you'll get a prompt asking you if you want to go there.
You can change the value in the function to anything you want, if you want the QR code to point to a different website.
Try this, and you'll the QR code changes. If you scan it with the camera on your phone, you'll see that it takes you to that website.
To see your QR Code live, you'll want to host the files locally. You can do this easily using Python's http server.
From within your project directory, run this command to start the server:
python3 -m http.server
Normally, this will host your files on port 8000, and will serve up index.html by default.
Go to localhost:8000 in your browser and you should see your QR code, displayed as the only item on the page.
That's how you create a QR Code in JavaScript. Feel free to read the QRious docs and experiment with different settings to learn more.