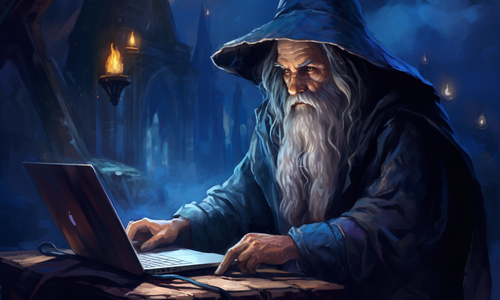
JavaScript POST Request with Example Code
Explanation of A Post Request
A POST request is a type of HTTP request.
You use a POST request when you want to send data to a server, typically so you can save it in a database.
A common use case would be: you have an app with an email catcher, a mini-form where the user can enter their email to be added to your email list.
Using a POST request, you can send that data to your server, so you can save it and add the user permanently to your email list.
You use a POST request whenever you want to save data permanently.
If a user signs up for your app or creates a comment or even votes something up - for all those you need a POST request.
HTTP Methods
POST is one of types of HTTP methods.
The full list of HTTP methods is: GET, POST, PUT, HEAD, DELETE, PATCH, OPTIONS, CONNECT, and TRACE.
The first two methods listed, GET and POST, are the most important ones. You may never need to implement the others, but a JavaScript developer will at some point need to use GET and POST.
A GET request is great for fetching data, but it cannot submit data. A POST request can.
So knowing how to do a POST request is an important skill for any developer to have.
POST Request Example
To make our POST request, we'll use a library called Axios.
Axios describes itself as a promise-based HTTP client for the browser and node.js. We'll be using it with node.js.
Typically, you might make a POST request from a portion of your webpage, after the user has submitted their data.
For example, let's say we've requested the user's data in a typical form, and now need to upload it: this is the place for a POST request.
Here is the code for just such a case.
async function handleSubmit(event) {
event.preventDefault();
const response=await axios.post(
'https://x0x0example.execute-api.us-east-1.amazonaws.com/production',
{ message: values.name, name: values.message, email: values.email }
);
}
Given different fields, you can always adjust the code as needed.
Download The Code
You can find the code for a full submit form, including a POST request, on GitHub.
https://gist.github.com/julianeon/767e32fc069d0a1568683deb4dd31fb0
This code comes from this tutorial on creating JavaScript email submit forms.
Usually, when doing a POST request to a database or an API, you would need to do authentication, but that is omitted here.
You can download this code and reuse it under an MIT license.
So that's how you do a POST request in JavaScript.
Feel free to study the submit form code also, to understand every step of the process as explained above.