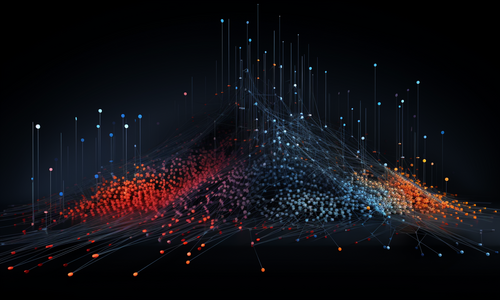
How to Get a Substring from a String In JavaScript
Using the Substring Method
You can get a substring from a string in JavaScript by using the substring method, which is available to use on any string.
The way the substring method works is with strings. A string is a primitive data type in JavaScript, used for sequences of characters.
You pass the method the indexes (the places in the string) you want it to extract, and it returns those, without destroying the original string.
Example Code for the Substring Method
Say that you define a string called name, using const or let:
const name="thomas";
You don't have to define the constant here explicitly as a string; this declaration will work for plain JavaScript.
You use the substring method on a string by adding it as a suffix to the end of the string.
name.substring(1,6);
Normally you tell the substring method where to start functioning and stop functioning, the "index" places in the string, where the counting starts at 0 and ends at the last position (exclusive).
You can generalize the method as follows:
string.substring(starting_place,ending_place)
Normally you use the substring method with two arguments, the starting place and the ending place of the substring you want (example.substring(5,15)).
If you only pass one argument, the substring method will still work, and will supply its own ending place, the end of the string (example.substring(5)).
Using the Substring Method with a Starting and Ending Index
For the string called name, defined to be "thomas", using a start and stop point of 1 and 4, you would expect the resulting substring to be "hom".
let piece=name.substring(1,4);
console.log(piece);
If you use console.log to print out this substring, you will see that it returns the substring "hom".
hom
Using the Substring Method with Only a Starting Index
You can also use the substring method with only a starting index.
It will then take the substring starting at the index you passed, and ending at the end of the string.
let simpler=name.substring(3);
console.log(simpler);
If you use console.log to print out this substring, you will see that it returns the substring "mas".
mas