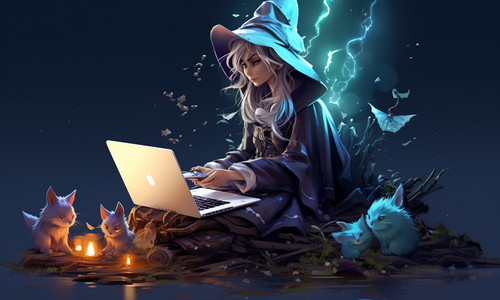
JavaScript GET Request with Example Code
Definition
A GET request is a fundamental HTTP request made using the standard HTTP method GET. This type of request is used when you need to retrieve data from a server. Here's a more detailed explanation of GET requests and their role in web development:
- Retrieving Data: GET requests are employed when you want to fetch data from a server. This could be data like a list of users, product names, comments stored in a database, or any information accessible via a server's API.
- Simplicity: Making a GET request in a JavaScript web page is straightforward and can often be accomplished in just a few lines of JavaScript code. One common method for making GET requests in JavaScript is using the `fetch` method.
- Example Result: The result of a JavaScript GET request is typically data that can be displayed on a standalone HTML page. This data is retrieved from the server and can be used for various purposes in your web application.
Example GET Request
You can see the result of a JavaScript GET request in action by visiting this URL:
JavaScript GET Request Example
In this example, a GET request is made to retrieve data, and the response is displayed on the HTML page.
GET Requests and APIs
GET requests are closely associated with APIs (Application Programming Interfaces). An API defines the methods and endpoints that your program or code can use to communicate with a server. It acts as a bridge between your application and the server, allowing you to request and receive data.
In the context of GET requests, APIs provide endpoints (URLs) that you can visit to retrieve specific data. One such example is the CoinDesk API, which provides cryptocurrency price data.
Here's an example CoinDesk API endpoint for fetching Bitcoin prices:
You can access this endpoint in a few lines of code to retrieve Bitcoin price data. The simplicity of GET requests makes them ideal for fetching data from various APIs across the web.
Additionally, you can even visit the API endpoint directly in your web browser to see the response data. Clicking on the link will display a text snippet containing the information you requested.
This is what many API's do: they dispense plain text, for you to style and display in your webpage.
Using API Data with CSS and Styling
The plain text version of API data is ugly. But with styling, it can look good. It can be made into presentable information people will like, and used in an app.
This is real-time information, so unlike something that's hard coded, it won't go out of date.
Here's that same information from the website above, styled nicely using CSS in this page, shown below.
...waiting
The Fetch Method
The code to display this is shown below. It's written for Svelte, which is the JavaScript framework this page is coded in.
I used the fetch method. It's simpler than an xlr request, an earlier way to do the same thing.
Fetch can be used anywhere xlr would have been used, so I would use fetch, the more common choice today.
const url='https://api.coindesk.com/v1/bpi/currentprice.json';
const fetchText = (async () => {
const response = await fetch(url);
return await response.json();
})()
Displaying the API Data On Your Page
The fetch method gets the data. Now you have to show it.
The way to show it differs between frameworks and JavaScript. But basically both use the 'await' keyword in an async (asynchronous) function.
The process is that the fetch request is made in code; when the response is received, that data is then displayed in the webpage.
Here's how it looks in plain JavaScript. You could put this in a plain HTML page and host it by itself and it would work.
<div class="box">
<div class="featured">
<p class="featured" id="banner">waiting...</p>
</div>
<div class="schmancy">
<div class="fancy">
<p>$<span id="usd">0</span> <span id="usd-description" style="float: right;" ></span></p>
<p>£<span id="gbp">0</span> <span id="gbp-description" style="float: right;" ></span></p>
<p>€<span id="eur">0</span> <span id="eur-description" style="float: right;" ></span></p>
</div>
</div>
</div>
<script>
const url='https://api.coindesk.com/v1/bpi/currentprice.json';
const fetchSet = (async () => {
const response = await fetch(url);
const data=await response.json();
document.getElementById("banner").innerHTML=data.time.updateduk.split("at")[0];
document.getElementById("usd").innerHTML=data.bpi.USD.rate;
document.getElementById("gbp").innerHTML=data.bpi.GBP.rate;
document.getElementById("eur").innerHTML=data.bpi.EUR.rate;
document.getElementById("usd-description").innerHTML=data.bpi.USD.description;
document.getElementById("gbp-description").innerHTML=data.bpi.GBP.description;
document.getElementById("eur-description").innerHTML=data.bpi.EUR.description;
});
fetchSet();
</script>
This is how it looks in Svelte.
{#await fetchText}
<p>...waiting</p>
{:then data}
<div class="featured">
<p>{data.time.updateduk.split("at")[0]}</p>
</div>
<div class="schmancy">
<div class="fancy">
<p>$ { data.bpi.USD.rate <span style="float: right;" } > { data.bpi.USD.description } </span></p>
<p>£ { data.bpi.GBP.rate <span style="float: right;" } > { data.bpi.GBP.description } </span></p>
<p>€ { data.bpi.EUR.rate <span style="float: right;" } > { data.bpi.EUR.description } </span></p>
</div>
</div>
{:catch error}
<p>An error occurred!</p>
{/await}
Despite the differences, you can still see the strong similarities.
Download The Code
Here's the code, in HTML & JavaScript, in a one page .html file.
You can download the file, rename it to .html, make changes to it, and see how that changes the page when you re-open the same file in your browser. It should appear like a normal webpage.
You can see the html page live as a standalone page here.
Here's the Svelte file, actually this page, as code. (Note: there are some small differences now that I've updated this page.)javascript-get-request-example.svelte
There's some CSS in there to make the animations, but that's presented without comment; you could remove all of that and it wouldn't change the core API/get request content.
Using This Code To Make Your Own GET Requests
So that's how you do a GET request.
It can get more complicated if you have to authenticate, which you do have to do for many API's, because they would get too many requests if there were no limits.
If the data returned is more complicated, or if you have to hit multiple endpoints, the amount of code you have to write can rapidly increase.
Many apps today would run automated jobs that will hit an API, store the data in a database, and then have their apps hit that database as often as they want (since there are no limits on that).
But for a simple webpage where you want to get API data directly and you're not worried about limits, this is how you do it.
Now that you know the procedure, which you can adapt it to your situation, and any APIs you want to fetch from.