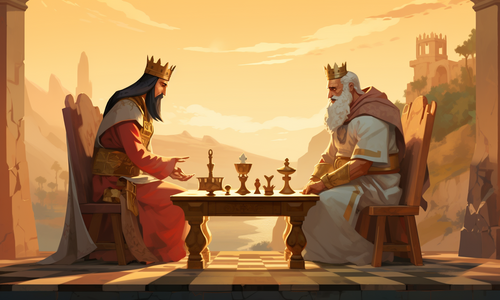
How to Create a Game Board Using JavaScript
The Elements of a JavaScript Game
JavaScript was originally developed to add small amounts of interactivity to a page. So it's a perfect tool for a game.
The biggest issue of all is gameplay: how your game will work. In a 'real' game this is where you'd spend the most time.
That's a distraction for a tutorial, so this game is purposefully simple. You can click boxes to change their color: that's the game.
While it would be normal for a project like this to distribute the code across several files, for this project they are all squeezed into one file, so you don't have to click between pages to understand what's going on.
This game is one HTML page, with the CSS and JavaScript also defined within the page.
Using HTML, CSS and JavaScript Together
The HTML page is the basic infrastructure for this page.
The CSS is used to define the game squares, the shape of the board and the individual squares.
The JavaScript is used for interactivity. When you click on a square, it changes its color. The gameplay itself is implemented in JavaScript.
With their powers combined, you have enough capability for a game-like experience.
This is what it will look like when you open it in your browser; clicking on the squares after you load the page will cause them to change color.
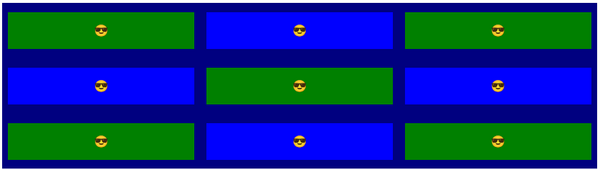
Walking Through the Code
This '1 page app' has a few pieces which interact together.
At the top, in the style section defining the CSS, the board game units are defined.
Using Flexbox, flexible units are defined which make it easy to create and space colored box units across the page.
Below that, you have the HTML, with the JavaScript inserted through the functions outerColor() and innerColor(), which activate when the user clicks on them.
Finally, in the section bookended by the script tag, you have the gameplay.
Using queryselector, you can select css elements - here, game board squares - and act on them.
For this game, we change their color, which causes the gameboard to blink, in different sections.
<!DOCTYPE html>
<html>
<head>
<title>Blinking Lights Game</title>
<style>
.box {
display: flex;
background-color: #000080;
}
.column {
display: flex;
justify-content: center;
flex: 1;
background-color: #e1c699;
margin-left: 1vw;
margin-right: 1vw;
margin-top: 1vh;
margin-bottom: 1vh;
text-align: center;
}
</style>
</head>
<body>
<div class="box">
<div onclick="outerColor('red')" class="column outerBox">
<p>😎</p>
</div>
<div onclick="innerColor('yellow')" class="column innerBox">
<p>😎</p>
</div>
<div onclick="outerColor('red')" class="column outerBox">
<p>😎</p>
</div>
</div>
<div class="box">
<div onclick="innerColor('blue')" class="column innerBox">
<p>😎</p>
</div>
<div onclick="outerColor('green')" class="column outerBox">
<p>😎</p>
</div>
<div onclick="innerColor('blue')" class="column innerBox">
<p>😎</p>
</div>
</div>
<div class="box">
<div onclick="outerColor('red')" class="column outerBox">
<p>😎</p>
</div>
<div onclick="innerColor('yellow')" class="column innerBox">
<p>😎</p>
</div>
<div onclick="outerColor('red')" class="column outerBox">
<p>😎</p>
</div>
</div>
<script>
function AddItem(boxClass) {
var boxChosen = document.querySelectorAll(".box ." + boxClass);
return boxChosen
}
const innerElements = AddItem("innerBox")
function innerColor(name) {
for (let i = 0; i < innerElements.length; i++) {
innerElements[i].style.backgroundColor = name
}
}
const outerElements = AddItem("outerBox")
function outerColor(name) {
for (let i = 0; i < outerElements.length; i++) {
outerElements[i].style.backgroundColor = name
}
}
</script>
</body>
</html>
Using the Code
You can run this app by copying the code into a file, labeling it index.html, and then opening it in your browser.
You should now see the app start up. Click on the boxes to make them change color.
And that's how you make a simple JavaScript board game.
Feel free to add to the JavaScript or add new functions to explore new capabilities you can add.