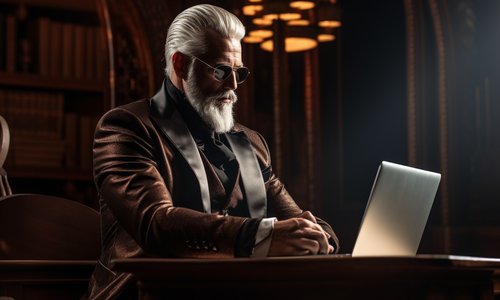
How to Calculate Compound Interest Using JavaScript
Compound Interest Formula
You can calculate compound interest easily in JavaScript.
The full formula for compound interest is:
A=P*(1+(r/n))^n*t
Where:
A=answer
P=initial balance (principal)
r=interest rate
n=number of times applied per unit time
t=number of units of time
We'll assume the interest compounds yearly (so n=1).
This gives us a simplified formula for compound interest, which looks like:
A=P*(1+r)^t
Formula Implementation Using a For Loop
Here is an implementation of that formula, written to be simple and clear, showing the principal, amount, rate, and calculation by year.
let amount;
let rate = .05;
let principal = 1000.0;
for ( let year = 1; year <= 10; year++ )
{
amount = principal * Math.pow(1 + rate, year);
console.log(year, amount);
}
Here is the same formula, where years are filled in an array from 0 through 10 (so fillrange, the array, looks like [0,1,2,3,4,5,6,7,8,9]).
const fillrange=[...Array(11).keys()];
for (const i in fillrange) {
amount = principal * Math.pow(1 + rate, i);
console.log(i, amount);
}
Alternative Implementations
Here is a very short version of finding the compound interest rate using map.
const result=fillrange.map((x)=>principal*Math.pow(1+rate,x));
console.log(result);
Here is another way to calculate compound interest, using the reduce method.
const amount_final=fillrange.reduce((previous,current)=>previous*(1+rate),principal);
console.log(amount_final);