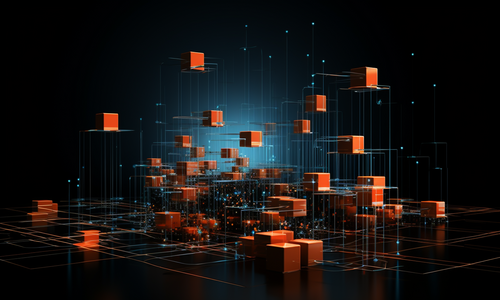
Arrays in JavaScript: From 1D to 3D Arrays
Introduction
Arrays are the cornerstone of data structures in JavaScript, providing a flexible way to store and manage ordered lists of values.
They serve as the foundation in many apps for the organization and processing of data. As a JS developer, you must know how to work with them.
In this blog post, we'll embark on a journey through the world of arrays, exploring the basics of 1D arrays and diving deeper into the multidimensional realms of 2D and 3D arrays.
Whether you're a beginner or looking to expand your understanding of arrays, this guide will help you grasp the basics of array dimension and array manipulation.
What Is An Array
An array is an ordered list of values; the values inside it are called the elements of the array. Every array element has a number, an index, you can use to access it.
You can define the elements of an array between square brackets, like so:
const example_array=[1,2,4,8,16];
For the following array examples, I'll be using strings (basically letters and words), though the array elements could be anything.
You can think of the dimension of an array as the number of indexes, or indices, you need to name a value of that array.
Think of dimension in geometry: 1d, 2d, 3d. We have the same thing with arrays.
1D Arrays
A 1d array, or a 1 dimensional array, is the default array you think of when presented with an array.
Here is a simple example, of length 4, expressed in the form of an array literal (where all the elements of the array are shown between square brackets).
const arr=["hey","man","nice","shot"];
2D Arrays
A 2D array is an array of arrays, where each array element contains its own array.
This 2d array is created using the Array() constructor, with several index values being filled with array literals. The array arr has 3 elements, and each element has its own array, of lengths 3, 5 and 2.
let arr=new Array();
arr[0]=["h","e","y"];
arr[1]=["h","e","l","l","o"];
arr[2]=["h","i"];
You can access its elements by specifying the array index number you need. The example below would access the [1][1] element and print the character 'e' to the console.
console.log(arr[1][1]);
3D Arrays
If a 1d array is a regular array, and a 2d array is an array of arrays, a 3d array adds one more layer: it's an array of arrays of arrays.
To create it, you can define each component javascript array element manually, and then combine those component arrays with elements that are also arrays into a new array. (That's a lot of arrays!)
let arr0=new Array();
arr0[0]=["h","e","y"];
arr0[1]=["h","e","l","l","o"];
arr0[2]=["h","i"];
let arr1=new Array();
arr1[0]=["to","the","team"];
arr1[1]=["chicken","beef","fish","lamb"];
let arr=[arr0,arr1];
console.log(arr[1][0][2]);
This code would access the [1][0][2] array element and print the unique string 'team' to the console.