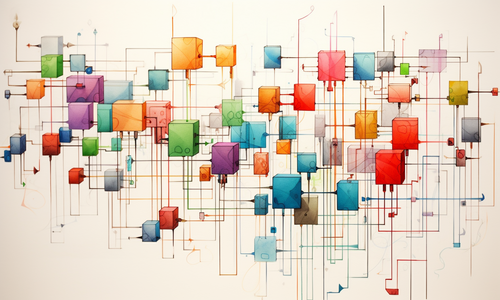
Variables in JavaScript
Introduction
Variables are the building blocks of any programming language. As with any language, you'll be working with them so often it pays to learn them well.
In JavaScript, variables allow you to store and manipulate data. You declare them and then you use them. It may seem obvious, but the details are important.
In this blog post, we'll dive deep into the world of variables in JavaScript, exploring their types, scope, naming conventions, and best practices.
Declaring Variables
In JavaScript, variables are declared using three different keywords: `var`, `let`, and `const`.
Each has its own characteristics, and choosing the right one depends on your specific use case.
var (Function-Scoped)
"var" is the oldest way to declare variables in JavaScript. It has function-level scope, which means the variable is accessible anywhere within the function it's declared in, but not necessarily within blocks (e.g., loops or conditional statements).
function exampleFunction() {
var x = 10;
if (true) {
var y = 20; // y is accessible here
}
console.log(x); // 10
console.log(y); // 20
}
let (Block-Scoped)
"let" was introduced in ECMAScript 6 (ES6) and provides block-level scope. This means a variable declared with `let` is confined to the block in which it is defined, such as loops or conditional statements.
function exampleFunction() {
let x = 10;
if (true) {
let y = 20; // y is only accessible within this block
}
console.log(x); // 10
console.log(y); // ReferenceError: y is not defined
}
const (Block-Scoped Constants)
"const" is also introduced in ES6 and is used for declaring constants. Once assigned, the value of a `const` variable cannot be changed.
const PI = 3.14159265359;
PI = 4; // Error: Assignment to constant variable
Variable Naming and Conventions
When naming variables in JavaScript, it's essential to follow best practices for clarity and maintainability:
- Use descriptive names: Choose names that reflect the purpose of the variable.
- Follow a consistent naming convention: Common conventions include camelCase (e.g., myVarName) for variables and PascalCase (e.g., MyClassName) for classes.
- Avoid reserved words: Don't use JavaScript reserved words (e.g., if, function, while) as variable names. However, you can use them if they're part of compound words (functionBuyNow).
- Be case-sensitive: JavaScript variable names are case-sensitive (myVariable is different from myvariable).
Global vs. Local Scope
Variables can have global or local scope.
Global variables are declared outside of any function or block and are accessible throughout the entire script, which can lead to unintended consequences.
Local variables are confined to the function or block where they are declared, which is generally a safer practice.
var globalVar = "I'm global";
function exampleFunction() {
var localVar = "I'm local";
console.log(globalVar); // Accessible
console.log(localVar); // Accessible
}
console.log(globalVar); // Accessible
console.log(localVar); // ReferenceError: localVar is not defined
Conclusion
Variables are a fundamental concept in JavaScript, allowing you to store and manipulate data effectively.
Understanding variable types, scope, naming conventions, and best practices is crucial for writing clean, maintainable, and error-free code.
Hopefully, this article has given you a good start. Try writing your own programs and experimenting with the ideas explained here, to get hands-on knowledge.
Every journey begins with a first step, and for JavaScript, learning about variables is a good place to begin.
Happy coding!