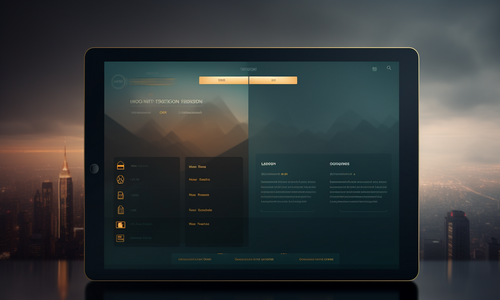
The DOM in JavaScript
Introduction
The Document Object Model (DOM) is the foundation of web development in JavaScript. It provides a structured representation of a web page's elements and allows developers to manipulate, traverse, and interact with these elements dynamically.
In this blog post, we'll cover the DOM in JavaScript, exploring its core concepts, manipulation techniques, event handling, and best practices.
What Is the DOM?
The Document Object Model (DOM) is a programming interface for web documents. It represents a structured tree-like hierarchy of HTML and XML elements on a web page.
In essence, the DOM allows JavaScript to interact with and manipulate the content and structure of a web page in real-time.
Accessing the DOM
You can access the DOM in JavaScript using the global `document` object. This object represents the entire web page and provides methods and properties to manipulate and interact with its elements.
Selecting Elements
You can select elements from the DOM using various methods, including:
- getElementById(): Selects an element by its unique `id` attribute.
- getElementsByClassName(): Retrieves elements by their class names.
- getElementsByTagName(): Selects elements by their tag name.
- querySelector() and querySelectorAll(): Use CSS-style selectors to select elements.
// Selecting an element by ID
const elementById = document.getElementById("myElementId");
// Selecting elements by class name
const elementsByClass = document.getElementsByClassName("myClassName");
// Selecting elements by tag name
const elementsByTag = document.getElementsByTagName("div");
// Using querySelector to select the first matching element
const firstElement = document.querySelector(".myClass");
Modifying the DOM
Once you've selected elements, you can modify their content, attributes, and structure. Common DOM manipulation methods include:
- innerHTML and textContent: Modify element content.
- setAttribute() and removeAttribute(): Change or remove element attributes.
- appendChild(), removeChild(), and replaceChild(): Add, remove, or replace child elements.
- createElement(), createTextNode(), and cloneNode(): Create new elements or clones.
// Modifying element content
elementById.innerHTML = "New content";
// Modifying element attributes
elementById.setAttribute("data-custom", "value");
// Appending a new child element
const newElement = document.createElement("p");
newElement.textContent = "This is a new paragraph.";
elementById.appendChild(newElement);
Event Handling
The DOM allows you to respond to user interactions by adding event listeners to elements.
Event listeners can detect and respond to events such as clicks, keypresses, and mouse movements.
// Adding a click event listener
elementById.addEventListener("click", function() {
alert("Element clicked!");
});
Traversing the DOM
Traversing the DOM involves navigating between elements.
You can move up and down the DOM tree using properties like `parentNode`, `childNodes`, `nextSibling`, and `previousSibling`.
The DOM provides numerous methods for efficient traversal, such as `querySelectorAll()` and `nextElementSibling`.
// Traversing the DOM to find the next sibling element
const nextSibling = elementById.nextElementSibling;
Best Practices
To maintain clean and efficient code when working with the DOM, consider these best practices:
1. Minimize DOM manipulation: Perform batch operations instead of multiple individual operations to minimize layout and rendering performance overhead.
2. Use event delegation: Attach event listeners to a common ancestor to handle events for multiple elements efficiently.
3. Cache DOM references: Store frequently accessed elements in variables to reduce repetitive DOM queries.
4. Use modern DOM APIs: Embrace newer DOM APIs like `querySelector()` and `addEventListener()` for cleaner and more expressive code.
5. Avoid global scope pollution: Limit the number of global variables and functions to prevent naming conflicts and potential issues.
Conclusion
The Document Object Model (DOM) is crucial for web development in JavaScript. The use of the DOM enables dynamic and interactive web applications.
By mastering DOM manipulation, understanding traversal techniques, and following best practices, you can create engaging user interfaces - which is what JavaScript was made to do.
As you continue to learn web development, set aside some time to learn more about manipulating the DOM through JavaScript. It'll make you a better developer and more effective at creating performant dynamic experiences.
You'll never run of things to learn about in JS, but the DOM is one of the more important ones. Study it. You'll be glad you did.