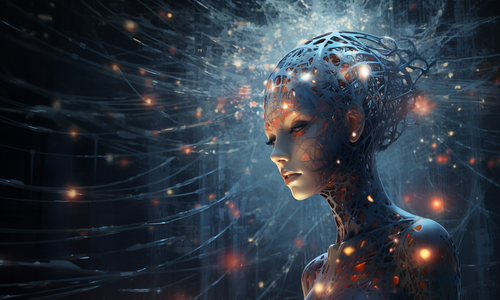
Making A Simple Neural Net In JavaScript
With AI being everywhere today, you've surely heard the phrase 'neural net.'
It turns out that, by using a library and following some relatively simple steps, you can create your own neural net too.
While it's only a toy, it's still useful for demonstrating how it's done and, at the library code level, exploring the concepts involved.
What Is A Neural Net
A neural net, or artificial neural net (ANN), is a term used in computing for machine learning systems that 'learn' using networks modeled after biological brains.
In a living brain, millions of neurons communicate with each other through signals distributed throughout the system. In a neural net, connected nodes 'communicate' with each other through math. You can use this output to detect patterns and solve problems.
By setting up your own, you can gain some insights into how it works too.
How to Make a Neural Net in JavaScript
To use our neural net, we're going to import a JavaScript library called brain.js.
Brain.js describes itself as a GPU accelerated neural network for JavaScript that is meant to be simple, fast and easy to use.
If you want to dive deeper into this and have more control over your options, you can try synaptic.js. For our purposes, brain.js is perfect, for its convenience, ease of use, and beginner friendliness.
Start by creating a new directory. I'll call mine neuralnet.
mkdir neuralnet
From within the directory, initialize a new npm project.
npm init -y
Install the brain.js library with the npm install command.
npm install --save brain
Now that we have the necessary tools, we'll write the JavaScript code to use the brain.js library.
We're going to lightly modify an example from the brain.js examples directory - writing a children's book.
https://brain.js.org/#/examples
In this example, you train the neural network with sentences, then ask it to predict the next word, based on the training set you gave it.
To run this example, create a file named net.js, and put this inside it.
const brain=require('brain.js');
const trainingData = [
'Jane saw Doug.',
'Doug saw Jane.',
'Spot saw Doug and Jane looking at each other.',
'It was love at first sight, and Spot had a frontrow seat. It was a very special moment for all.'
];
const lstm = new brain.recurrent.LSTM();
const result = lstm.train(trainingData, {
iterations: 1500,
log: details => console.log(details),
errorThresh: 0.011
});
const run1 = lstm.run('Jane');
const run2 = lstm.run('Doug and Spot');
const run3 = lstm.run('Spot loves');
const run4 = lstm.run('Jane loves');
console.log('run 1: Jane' + run1);
console.log('run 2: Doug and Spot' + run2);
console.log('run 3: Spot loves ' + run3);
console.log('run 4: Jane loves ' + run4);
}
If you try to run this code using a .js extension, you'll receive an error about 'running outside a module.'
So rename the file net.cjs and run it using the node net.cjs command.
node net.cjs
You should see output which looks like this, which shows the code is working.
...
iterations: 300, training error: 0.01124519244889394
iterations: 310, training error: 0.011074903969527324
run 1: Jane and Spot saw Doug and Jane looking at each other.
run 2: Doug and Spot had a frontrow seat. It was a very special moment for all.
run 3: Spot loves at first sight, and Spot had a frontrow seat.
It was a very special moment for all.
run 4: Jane loves . It was love at first sight, and Spot had a frontrow seat.
It was a very special moment for all.
Conclusion
Now you know how to set up and use a neural net using JavaScript, a very simple one.
Hopefully, this will be the start of your learning journey. Now it's up to you to learn more about this deep and important topic.
If you want to learn more, consider watching this video tutorial on setting up and running a neural net from scratch on scrimba.com.
Beyond that, you could also consider an online course or certificate, like this one, "IBM Machine Learning Professional Certificate". It covers ANN's, or artificial neural nets.
While I don't have a crystal ball, I can absolutely guarantee that AI will be big part of the future of computing, and a certificate like this can only help.
Happy learnings!