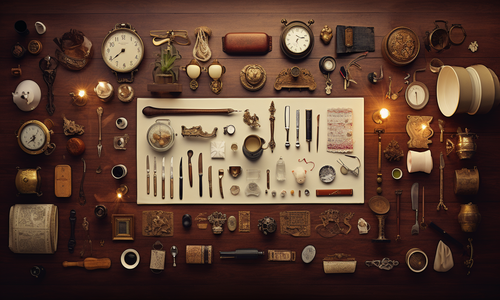
Sorting JavaScript Arrays of Objects by Key
If you want to sort an array of objects by a specific key using JavaScript, you can do it by using these methods.
Sorting an Array by a Specific Key
The primary method most people use is the sort method available on arrays.
To sort an array of objects by a specific key, you pass a comparator function to the sort method.
The comparator function compares two objects and determines their order in the sorted array.
const arr = [
{ id: 3, name: 'David' },
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Charlie' }
];
arr.sort((a, b) => a.id - b.id);
console.log(arr);
// Output:
// [
// { id: 1, name: 'Alice' },
// { id: 2, name: 'Charlie' },
// { id: 3, name: 'David' }
// ]
In this example, we're sorting by the id key.
The comparator function is (a, b) => a.id - b.id, which sorts in ascending order based on the id.
Or, even more simply:
array.sort(function(a, b) {
return a[key] - b[key];
});
Where array is the array to be sorted and key is the key to sort on.
If you wanted to sort in descending order, you would switch the places of a and b.
Using a Generic Function to Sort by Any Key
If you find yourself needing to sort arrays of objects by various keys, it might be useful to create a more generic function.
In this example, the sortByKey function has been written in such a way that it can sort an array of objects by any specified key.
function sortByKey(array, key) {
return array.sort((a, b) => {
if (a[key] < b[key]) return -1;
if (a[key] > b[key]) return 1;
return 0;
});
}
const arr = [
{ id: 3, name: 'David' },
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Charlie' }
]
const sortedArr = sortByKey(arr, 'name');
console.log(sortedArr);
// Output:
// [
// { id: 1, name: 'Alice' },
// { id: 2, name: 'Charlie' },
// { id: 3, name: 'David' }
// ]
Sorting an Array by Multiple Keys
In JavaScript, if you want to sort an array of objects by multiple keys, you can use the `sort()` function in combination with a comparator function. Here's how.
1. Use the `sort()` method on the array.
2. In the comparator function, compare the values of the first key.
3. If the values of the first key are equal, compare the values of the next key, and so on.
Here's an example:
Imagine you have the following array of objects, sorted first by `age` and then by `name`.
const users = [
{ name: 'John', age: 25 },
{ name: 'Doe', age: 25 },
{ name: 'Anna', age: 22 },
{ name: 'Chris', age: 22 },
{ name: 'Mike', age: 30 }
];
users.sort((a, b) => {
if (a.age !== b.age) {
return a.age - b.age; // Sort by age first
} else {
return a.name.localeCompare(b.name); // If ages are the same, sort by name
}
});
console.log(users);
This will output:
[
{ name: 'Anna', age: 22 },
{ name: 'Chris', age: 22 },
{ name: 'Doe', age: 25 },
{ name: 'John', age: 25 },
{ name: 'Mike', age: 30 }
]
Notice how users with the same age are sorted by their name.
You can extend this approach to include more keys if needed.
And that's how it's done.
Summary
To recap:
1. To sort an array by a specific key, pass a comparator function to the sort method.
2. To sort an array by any key, generalize your method to its own function.
3. To sort an array by multiple keys, you can use the sort() function in combination with a comparator function.
May all your sorts always return the answer you seek. 🙏
Thanks for reading!