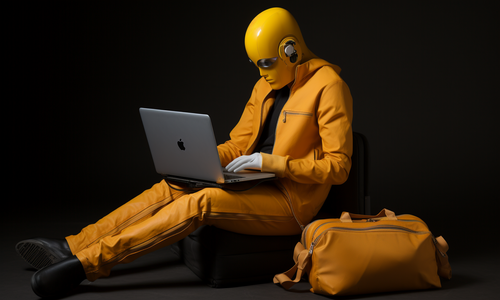
JavaScript Crash Course: The Core Features
Introduction
JavaScript, commonly abbreviated as JS, is the widely used programming language that powers the web. With JavaScript, you can add interactivity, functionality, and dynamism to your web pages.
In this JavaScript crash course, we'll dive into the language's core features to give you a solid understanding of how it works.
Variables and Data Types
JavaScript is a dynamically typed language. In JS you don't need to declare the data type of a variable explicitly (which isn't always a good thing - see TypeScript for more).
You can declare variables using the var, let, or const keywords.
- var (function-scoped): Used for variable declarations in older versions of JavaScript.
- let (block-scoped): Introduced in ES6, it's now the preferred way to declare variables.
- const (block-scoped): Used to declare constants; the value cannot be reassigned.
JavaScript supports various data types, including:
- Numbers (e.g., 42, 3.14)
- Strings (e.g., "Hello, World!")
- Booleans (e.g., true, false)
- Arrays (e.g., [1, 2, 3])
- Objects (e.g., { name: "John", age: 30 })
- Null and Undefined
Operators
JavaScript supports a wide range of operators for performing operations on data:
- Arithmetic operators (+, -, *, /, %)
- Comparison operators (==, !=, ===, !==, <, >, <=, >=)
- Logical operators (&&, ||, !)
- Assignment operators (=, +=, -=)
- Ternary operator (condition ? trueExpression : falseExpression)
Control Flow
JavaScript offers various control flow statements to make decisions and repeat actions:
- if statement: Executes code based on a condition.
- else and else if clauses: Provide alternative code paths.
- switch statement: Allows multi-case selection.
- for loop: Repeats code a specified number of times.
- while and do...while loops: Execute code while a condition is true.
- break and continue statements: Control loop flow.
Functions
Functions in JavaScript are reusable blocks of code that can be called with arguments. You can define functions using the function keyword:
function greet(name) {
console.log(Hello, $ {name}!);
}
Arrow functions, introduced in ES6, offer a more concise way to define functions:
const greet = (name) => {
console.log(Hello, $ {name}!);
};
Objects and Classes
JavaScript is an object-oriented language, and objects play a crucial role.
You can create objects using object literals or constructor functions.
ES6 introduced classes, making object-oriented programming more accessible:
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
sayHello() {
console.log(Hello, my name is $ {this.name}.);
}
}
const person = new Person("John", 30);
person.sayHello();
6. Events and Event Handling
In web development, JavaScript is commonly used to respond to user interactions and events.
You can add event listeners to HTML elements to trigger JavaScript functions when events occur:
const button = document.getElementById("myButton");
button.addEventListener("click", () => {
console.log("Button clicked!");
});
Conclusion
JavaScript is a powerful and versatile language that can be used for web development, server-side scripting, and more.
This crash course has hopefully given you a grasp of the core features of JS.
With this knowledge, starting with the concepts you see here, you can start exploring and building your own interactive web applications.
As you continue your journey, don't forget to practice, practice, and practice some more on your way to becoming a truly great developer.
Happy coding!