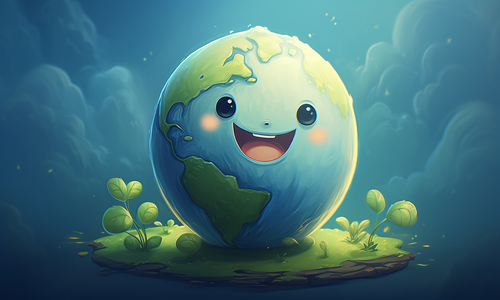
Hello World in JavaScript
Introduction
The "Hello World" program is a tradition when you're learning a new programming language. At JavaScriptPage, we respect tradition.
While it's typically simple text (as with the node.js one-liner console.log('hello world')), we can make it more interesting by adding dynamic effects using JavaScript and CSS.
In this tutorial, we'll create a "Hello World" message that gracefully fades in and out using JavaScript.
Prerequisites
Before we dive into the code, make sure you have the following:
1. A text editor or integrated development environment (IDE) of your choice.
2. A web browser to test your code.
Let's get started!
HTML Setup
First, create an HTML file (e.g., 'index.html') with this basic structure:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Hello World Fading Text</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="container">
<h1 id="message">Hello, World!</h1>
</div>
<script src="script.js"></script>
</body>
</html>
In this HTML page, we have a 'div' with the class "container" containing an 'h1' element with the id "message."
We'll use JavaScript to manipulate the text within this 'h1' element.
CSS Styles (styles.css)
To style our "Hello World" message and apply the fading effect, create a CSS file (e.g., 'styles.css') with the following content:
body {
font-family: Arial, sans-serif;
background-color: #f2f2f2;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
}
.container {
text-align: center;
}
#message {
font-size: 3rem;
color: #333;
opacity: 0;
transition: opacity 1s ease-in-out;
}
This CSS code styles the "Hello, World!" message and initially sets its opacity to 0 to make it invisible.
JavaScript Logic (script.js)
Now, create a JavaScript file (e.g., 'script.js') to add the fading effect:
const message = document.getElementById("message");
function fadeIn() {
message.style.opacity = "1";
}
function fadeOut() {
message.style.opacity = "0";
}
function toggleFade() {
if (message.style.opacity === "1") {
fadeOut();
} else {
fadeIn();
}
}
setInterval(toggleFade, 2000);
// Toggle fade every 2 seconds
In this JavaScript code, we first select the 'h1' element with the id "message" using 'document.getElementById'.
We then define three functions: 'fadeIn', 'fadeOut', and 'toggleFade'.
- 'fadeIn' sets the opacity of the message to 1, making it fully visible.
- 'fadeOut' sets the opacity to 0, making it invisible.
- 'toggleFade' checks the current opacity state of the message and toggles between fading in and out.
Finally, we use 'setInterval' to call 'toggleFade' every 2 seconds (2000 milliseconds), creating the fading effect.
Conclusion
Congratulations! You've successfully created a dynamic "Hello World" message using JavaScript and HTML.
This simple project demonstrates the power of JavaScript in manipulating HTML elements and adding dynamic effects to your web pages.
Wasn't so hard, was it? Keep doing a project like this every day and you'll pick up JS in no time.
Feel free to customize this example to create even more engaging experiences.
Happy coding!