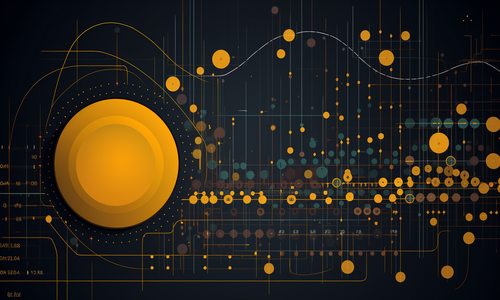
Functions in JavaScript
Introduction
Functions are the backbone of JavaScript programming. They enable you to encapsulate reusable blocks of code, making your programs more organized and efficient.
In this blog post, we'll explore functions in JavaScript, covering their syntax, types, parameters, return values, and best practices.
Defining Functions
In JavaScript, you can define functions using the "function" keyword, followed by a name, a list of parameters (if any), and the function body enclosed in curly braces {}. Here's a simple function that greets a user:
function greet(name) {
console.log('Hello, $ {name}!');
}
Function Types
JavaScript supports two primary types of functions: named functions and anonymous functions.
Named Functions
Named functions are declared with a name and can be used multiple times throughout your code.
function add(a, b) {
return a + b;
}
const result = add(3, 5);
// Calls the add function and stores the result in 'result'
Anonymous Functions
Anonymous functions, also known as function expressions, are defined without a name. They are often used as arguments for other functions or assigned to variables.
const subtract = function (a, b) {
return a - b;
};
const result = subtract(10, 4);
// Calls the anonymous function stored in 'subtract'
Parameters and Arguments
Parameters are placeholders for values that a function can accept. When you call a function, you provide actual values called arguments.
function multiply(a, b) {
return a * b;
}
const product = multiply(4, 6);
// 'a' is 4, 'b' is 6
Return Values
Functions can return values using the "return" statement. If a function doesn't explicitly return a value, it returns "undefined".
function divide(a, b) {
if (b === 0) {
console.error("Division by zero");
return; // Early return
}
return a / b;
}
const result = divide(8, 2);
// Returns 4
Function Best Practices
To write clean and maintainable code, consider the following best practices when working with functions in JavaScript:
1. Use descriptive names: Choose meaningful names for functions that indicate their purpose.
2. Keep functions focused: Functions should have a single responsibility. If a function becomes too large or complex, consider splitting it into smaller functions.
3. Document your functions: Use comments or JSDoc comments to describe the purpose of the function, its parameters, and return values.
4. Avoid global variables: Minimize the use of global variables within functions to prevent unintended side effects.
5. Use arrow functions (ES6): Arrow functions provide a concise syntax for defining functions, especially for short, one-liner functions.
const double = (x) => x * 2;
Conclusion
Functions are a fundamental building block of JavaScript, allowing you to structure and organize your code efficiently.
By understanding function types, parameters, return values, and following best practices, you'll be well-equipped to write clean, modular, and maintainable JavaScript code.
As you continue your journey in JavaScript development, functions will also help you "function" better. (Note: we are not professional comedians).
Happy coding!