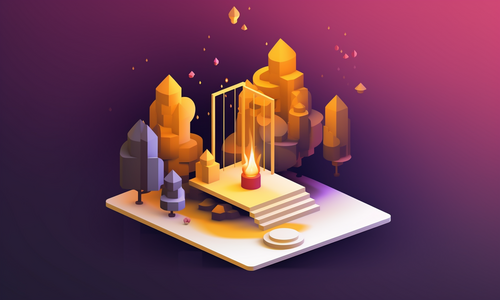
Events in JavaScript
Introduction
Events are at the heart of interactivity in web development. They enable you to create responsive and engaging user interfaces.
In JavaScript, events are essential for capturing user interactions and triggering corresponding actions.
In this blog post, we'll dig into the world of events in JavaScript, covering the basics, event handling, event types, and best practices.
Whether you're a noob at web development or a seasoned pro, understanding events is crucial for creating dynamic and interactive web applications.
Understanding Events
In the context of web development, events are occurrences or happenings triggered by users or the browser itself.
Examples of events include clicking a button, moving the mouse, typing on the keyboard, or resizing the browser window.
Event Handling
Event handling is the process of defining code that should execute in response to a specific event.
JavaScript provides a straightforward way to add event listeners to HTML elements.
Here's how you can attach an event listener to a button element:
const button = document.getElementById("myButton");
button.addEventListener("click", function() {
alert("Button clicked!");
});
In this example, we're adding a "click" event listener to the button element.
When the button is clicked, the anonymous function is executed, displaying an alert.
Common Event Types
JavaScript supports a wide range of event types.
Some of the most commonly used event types include:
- click: Occurs when a mouse click is detected on an element.
- mousedown and mouseup: Triggered when the mouse button is pressed down or released, respectively.
- mousemove: Fires as the mouse pointer moves within an element.
- keydown and keyup: Correspond to pressing and releasing a keyboard key.
- submit: Triggered when a form is submitted.
- focus and blur: Occur when an element gains or loses focus.
Event Object
When an event occurs, JavaScript provides an event object containing information about the event, such as the type, target element, mouse coordinates, and more.
You can access this event object as a parameter in your event handler function:
element.addEventListener("click", function(event) {
console.log(Event type: $ {event.type});
console.log(Target element: $ {event.target});
console.log(Mouse X-coordinate: $ {event.clientX});
console.log(Mouse Y-coordinate: $ {event.clientY});
});
Event Bubbling vs. Event Capturing
Events in JavaScript follow a propagation order, which can be either "bubbling" or "capturing."
By default, most events use the "bubbling" phase, where the event starts at the target element and bubbles up to the document root.
You can also use the "capturing" phase, where the event starts at the document root and trickles down to the target element.
To use capturing, set the third parameter of "addEventListener" to "true":
element.addEventListener("click", function() {
console.log("Event in capturing phase");
}, true);
Event Delegation
Event delegation is a powerful technique that involves attaching a single event listener to a common ancestor of multiple elements.
This allows you to efficiently handle events for elements dynamically added to or removed from the DOM.
It reduces the memory and performance overhead associated with attaching individual event listeners to each element.
document.getElementById("parentElement").addEventListener("click", function(event) {
if (event.target.classList.contains("clickable")) {
// Handle the click on a specific element with the "clickable" class.
}
});
Best Practices
To ensure clean and efficient event handling in your JavaScript code, consider the following best practices:
1. Separation of concerns: Keep your HTML, CSS, and JavaScript separate to promote code maintainability.
2. Unobtrusive JavaScript: Avoid inline event handlers in HTML (onclick, onmouseover, etc.) in favor of adding event listeners in JavaScript.
3. Use event delegation: For dynamically created elements, use event delegation to minimize the number of event listeners.
4. Optimize event handlers: Be mindful of performance when handling frequent events like `mousemove`. Consider throttling or debouncing event handlers.
5. Remove event listeners: When you no longer need an event listener, remove it using `removeEventListener` to prevent memory leaks.
element.removeEventListener("click", eventHandlerFunction);
<h2>Conclusion</h2>
Conclusion
Events are the cornerstone of interactive web development in JavaScript, allowing you to respond to user actions and create dynamic web applications.
By mastering event handling, understanding event types, and following best practices, you can build responsive and engaging user interfaces.
Plus, once you get good at using them, they're fun. Interactivity is fun. Never lose sight of the fun side of JavaScript development.
To learn more, try adding an event handler to your own code, and keep going from there.
And may your JavaScript programs, like your life as a developer, be eventful.