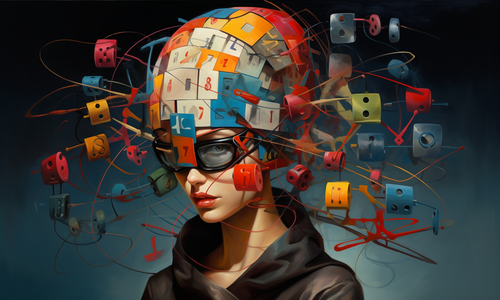
Conditionals in JavaScript
Introduction
When writing JavaScript code - or any code - one of the fundamental concepts you'll encounter is conditionals.
Conditionals are constructs allow you to make decisions and control the flow of your program based on certain conditions.
In this blog post, we'll explore various aspects of conditionals in JavaScript, from basic if statements to advanced techniques for handling complex logic.
The if Statement
The if statement is a foundational building block of conditionals in JavaScript. It allows you to execute a block of code if a specified condition evaluates to true.
if (condition) {
// Code to execute if the condition is true
}
Here's an example of using an if statement:
const temperature = 25;
if (temperature > 30) {
console.log("It's hot outside!");
}
The else Statement
The else statement complements the if statement by providing an alternative block of code to execute when the condition is false.
if (condition) {
// Code to execute if the condition is true
} else {
// Code to execute if the condition is false
}
const age = 17;
if (age >= 18) {
console.log("You are an adult.");
} else {
console.log("You are a minor.");
}
The else if Statement
For handling multiple conditions, you can use the else if statement. It allows you to test additional conditions if the previous ones are false.
if (condition1) {
// Code to execute if condition1 is true
} else if (condition2) {
// Code to execute if condition2 is true
} else {
// Code to execute if all conditions are false
}
const grade = 85;
if (grade >= 90) {
console.log("A");
} else if (grade >= 80) {
console.log("B");
} else if (grade >= 70) {
console.log("C");
} else {
console.log("D");
}
The switch Statement
The switch statement is an alternative to nested if and else if statements, particularly useful when you need to compare a single value against multiple possibilities.
switch (expression) {
case value1:
// Code to execute if expression matches value1
break;
case value2:
// Code to execute if expression matches value2
break;
default:
// Code to execute if no matches are found
}
const dayOfWeek = "Wednesday";
switch (dayOfWeek) {
case "Monday":
console.log("It's the start of the week.");
break;
case "Wednesday":
console.log("It's the middle of the week.");
break;
default:
console.log("It's another day of the week.");
}
Ternary Operator
The ternary operator (? :) offers a concise way to write conditional expressions in JavaScript. It's often used for simple conditions.
const result = condition ? valueIfTrue : valueIfFalse;Here's an example:
const age = 20;
const message = age >= 18 ? "You are an adult." : "You are a minor.";
console.log(message);
Truthy and Falsy Values
In JavaScript, values are not necessarily true or false; they can also be truthy or falsy. This can become important when an unexpected value causes an unexpected result, leading to a bug.
- Truthy Values: Values that evaluate to true in a Boolean context.
- Falsy Values: Values that evaluate to false in a Boolean context.
Here are some common falsy values:
- false
- 0
- "" (an empty string)
- null
- undefined
- NaN (Not-a-Number)
const value = "";
if (value) {
console.log("Truthy");
} else {
console.log("Falsy");
}
Logical Operators
Logical operators (&&, ||, !) allow you to combine conditions and create more complex logic in your conditionals.
- && (Logical AND): Returns true if both conditions are true.
- || (Logical OR): Returns true if at least one condition is true.
- ! (Logical NOT): Inverts the Boolean value of a condition.
Here's an example:
const temperature = 25;
const isSummer = true;
if (temperature > 30 && isSummer) {
console.log("It's a hot summer day!");
}
Best Practices
When working with conditionals in JavaScript, it's essential to follow best practices for writing clean and maintainable code:
- Use meaningful variable and function names to improve code readability.
- Properly indent your code to make the logic clear.
- Comment complex or non-obvious parts of your code.
- Avoid deeply nested conditionals; consider refactoring for better readability.
- Use consistent coding styles and conventions to enhance code maintainability.
Conclusion
Conditionals are a fundamental part of programming in JavaScript. They allow you to make decisions and create dynamic, responsive code.
By understanding the various conditional statements, truthy and falsy values, and logical operators, you'll be better equipped to write efficient and readable code in JavaScript.